Build your first Flutter mobile app

If you’re trying to get into mobile development, you’ve likely come across Flutter at some point.
Today I’m going to start a series of detailed blog posts on how to use flutter framework from basics to advance.
Starting with this post, you will learn how to use google flutter. here we will discuss basic concepts of google flutter. so, without wasting your time let’s start.
Essential Concept Before starting with the basics of Flutter.
Flutter is a cross-platform mobile application development framework created by Google. It is a set of technologies which allow the developers to build apps on multiple platforms with one code base.
The Flutter team is committed to using the mobile development platform to help developers create apps that will let users have a better experience. Given the extensive features and capabilities of Flutter, it makes sense that the framework has found itself in use in so many apps.
What is Dart?
Dart is a compiled language which is used in Flutter. Dart is similar to Object oriented programming languages such as Java. By combining the power of Dart and Flutter frameworks, developers can create app experiences that are much more sophisticated than those offered by most other mobile app development frameworks.
Before getting started with Flutter, we should know that Flutter is developed using Dart language.
Flutter also supports Android, iOS, web, and other platforms.
Examples of Flutter Apps
Here is an example of a complex app that I build using Flutter: Wi-Fi File Transfer
What is Flutter Architecture?
Underneath the hood, Flutter is built around three foundational libraries that provide the building blocks of its design:
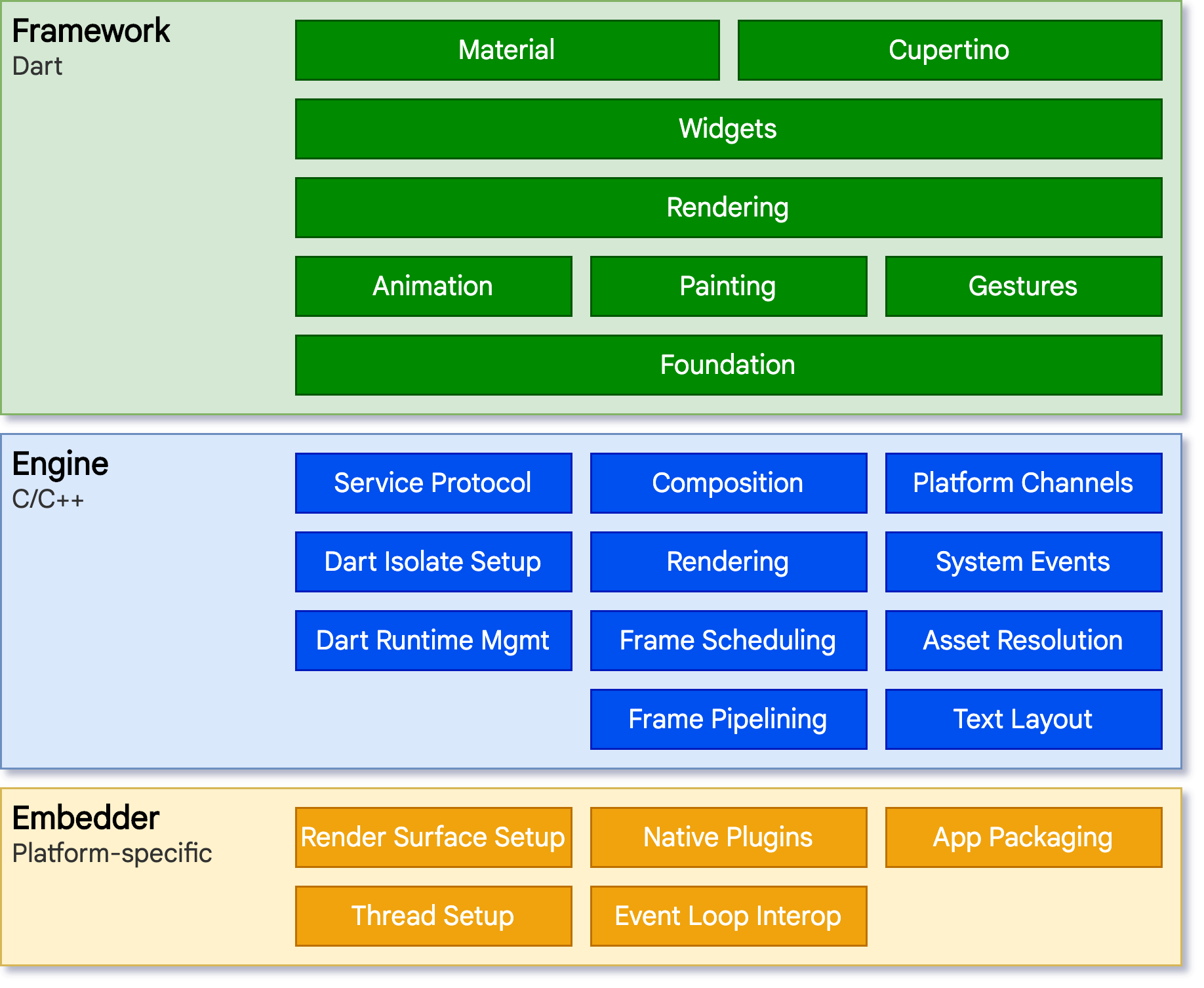
Each of these layers are completely replaceable by an alternative implementation. This approach is known as “isolate by default, communicate by choice”.
You can learn about each of these layers in great details in this link.
However, these are the main layers that you will be focusing on while building your application.
Application layer
The Application layer is where you write the code for your application. The code here is written in the Dart programming language.
App widget
A widget is an abstract widget. A widget is an object that has a layout and a set of properties for layout.
In the App widget, you declare the layout of your widget by giving it a layout object. You can also define the properties of the layout that you want to use, such as padding, margin, and textAlign. You can also define the properties that you want to use to style the widget, such as backgroundColor and elevation.
Widgets form a hierarchy based on composition. Each widget nests inside its parent and can receive context from the parent. This structure carries all the way up to the root widget (the container that hosts the Flutter app, typically MaterialApp or CupertinoApp), as this trivial example shows:
import 'package:flutter/material.dart';
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Container(
// Called before the widget is displayed on the screen.
// Appends to the existing context, if any, and creates
// a default context if none is provided.
child: ConstrainedBox(
// The nested widget to display.
constraints: BoxConstraints(minHeight: 200.0, minWidth: 200.0),
child: FadeInImage(
// The image to display, as an example.
image: NetworkImage('https://i.imgur.com/dexzkP4.jpg'),
placeholder: AssetImage('assets/images/loading.gif'),
fit: BoxFit.cover,
height: 200.0,
width: 200.0,
),
// As a result, the image appears at the top left corner of
// the screen.
),
);
}
}
In this example, the child widget (FadeInImage) is constrained by the parent widget (Container) and by the top-level
Install Flutter
By now you have basic understanding of what flutter is, let’s build your first application using flutter.
To get started, first you’ll need to setup your flutter development environment following this official link:
Let’s assume you’ve already set up the environment as follow:
$ flutter doctor
and your flutter doctor say that you are all good.
Now that you have the flutter SDK setup, you can start developing with flutter. In this tutorial we will build a Reddit client which is a moderately complex application and by doing so we can get familiar with many different Flutter features.
IntelliJ and Android Studio IDE
I personally use IntelliJ IDE and Android studio IDE for most of my developments but for Flutter you have other choices such as Visual Studio Code which has a great support for flutter development.
Android studio is an IDE from the Android developer tools suite, which allows the development and debugging of Android apps. It provides IDE, frameworks, SDK, device simulation and several more tools which make it easy to develop mobile apps.
Reddit Client App built on Flutter
I believe the best way to learn flutter, or any framework and programming language is to create an application with medium complexity. For this I’m going to walk you through a series of tutorials to build a practically complex flutter application to build your knowledge from beginner to advanced.
Use the flutter command line tools to create a new project: It is possible to pass other arguments to flutter create, such as the project name (pubspec.yml), the organization name, or to specify the programming language used for the native platform:
$flutter create --project-name reddit_client --org com.techprd --android-language kotlin --ios-language swift reddit-client
now go to the project folder
$ cd reddit-client
Attach an android phone or iPhone to your PC via a USB and make sure the developer mode is on. Now run the flutter application:
$ flutter run
Congratulations, you have successfully built your first flutter application.
reddit_client (this link opens in a new window) by techprd (this link opens in a new window)
A simple reddit client built on flutter
In the next tutorial we will add the basic features of our Reddit Client app.